はじまり
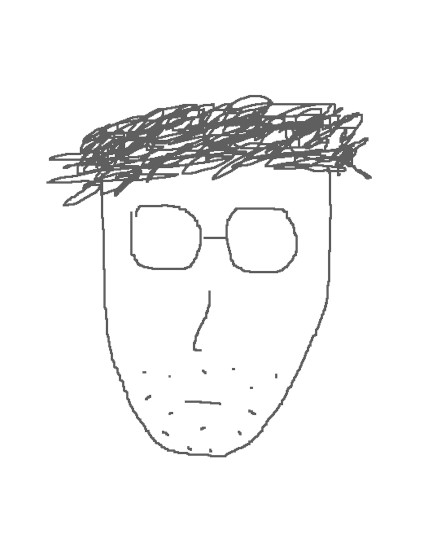
135ml
うーん、月曜から金曜まで一気通貫の予定として入力するとスケジューリングしにくいなあ・・・。日にちごとに予定をいじりたいのに・・・。
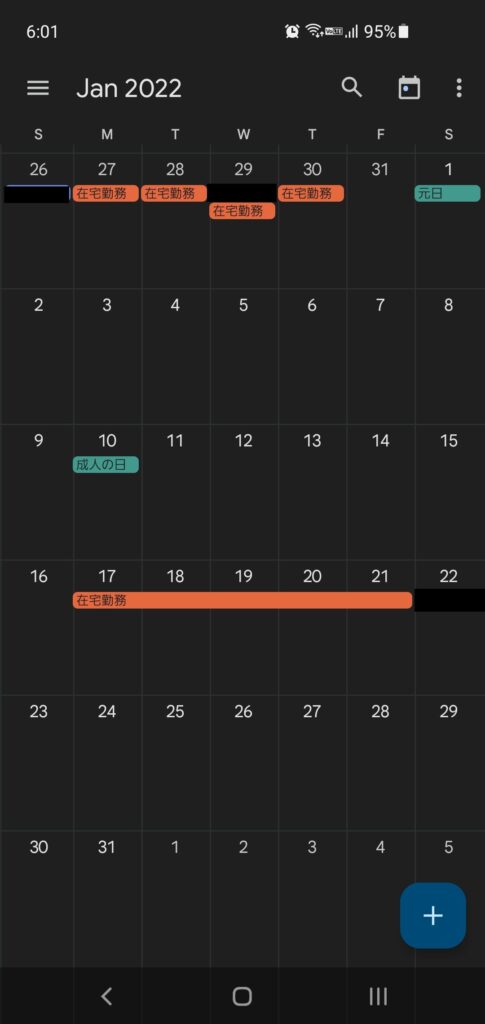
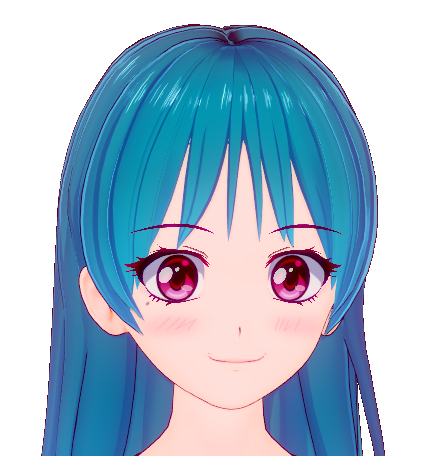
リサちゃん
おーい、ボンカレー食べるかー?
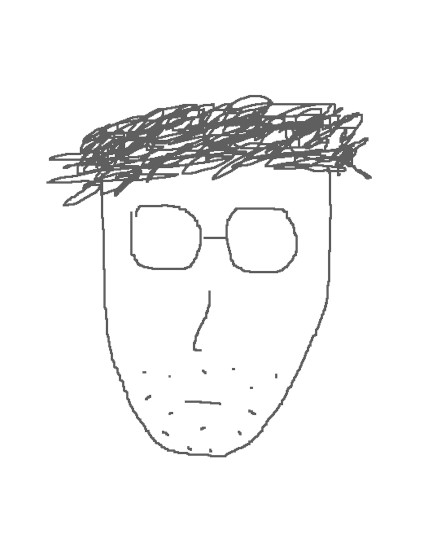
135ml
よし! 1日ずつ予定を打ち込めるスクリプトを作るぜ、俺は!
うおおおおお!!!
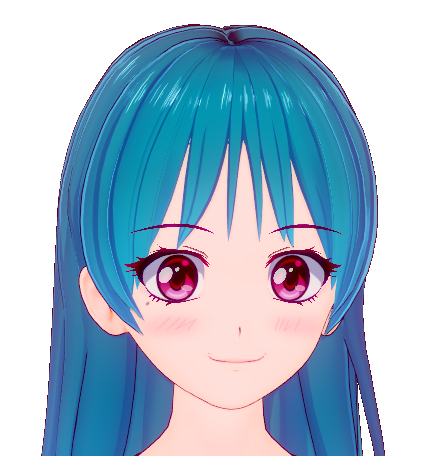
リサちゃん
おーい、ボンカレー食べるかー?
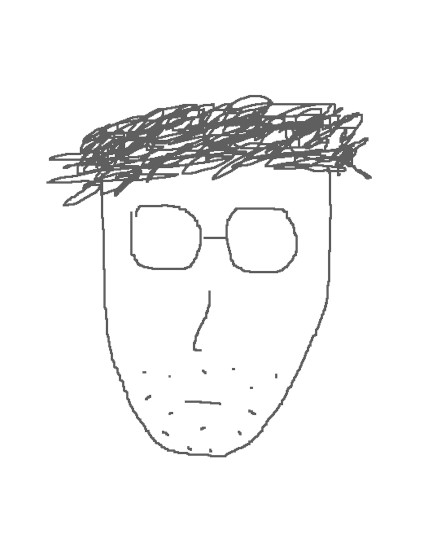
135ml
食べる!
ツールの紹介
ツールはこんな感じになっています。
- startDateに連続した予定期間の開始日を入力して、endDateに終了日を入れます。
- それと予定の「title」と「startTime(開始時刻)」と「endTime(終了時刻)」も入力します。
- 「color」は、下の「専用コード(color)」の方から予定の色にしたい色を決めて、「色番号」の数字の中から入力します。
「description」「location」「guests」「sendInvites」は任意項目です。
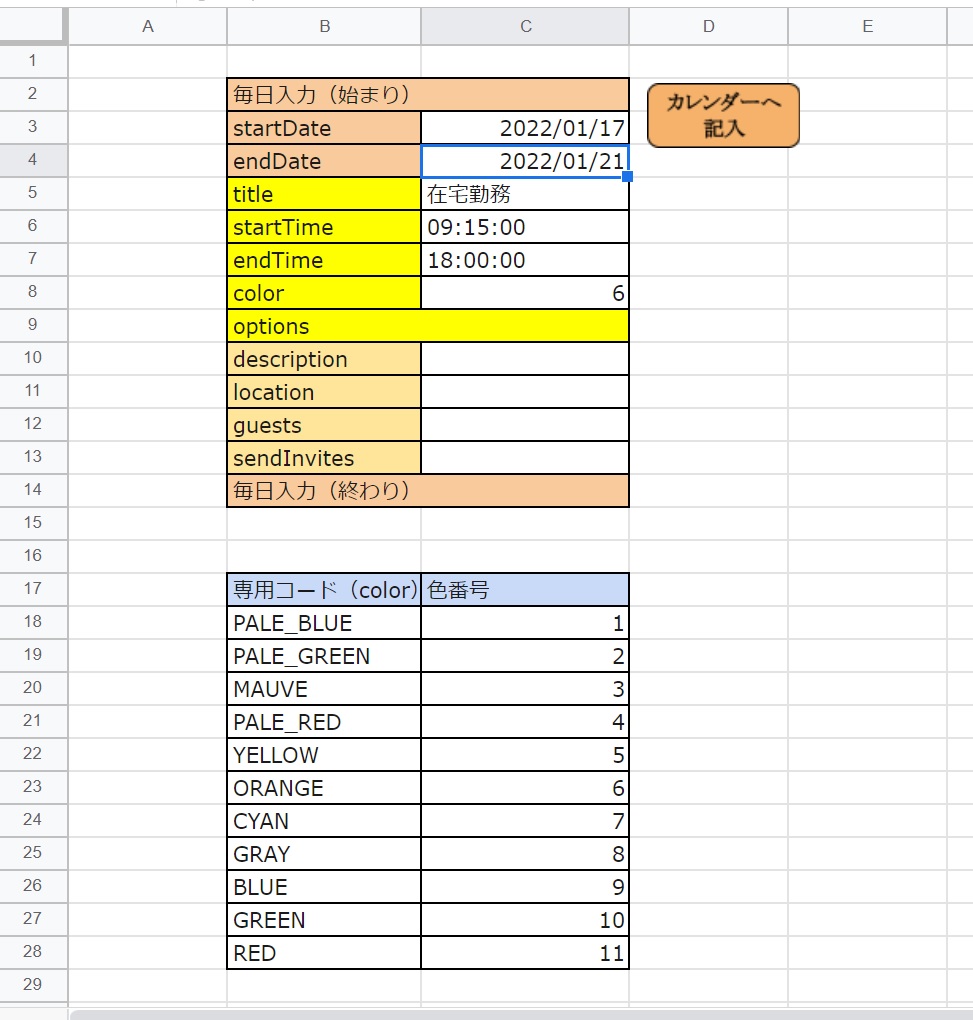
実際に
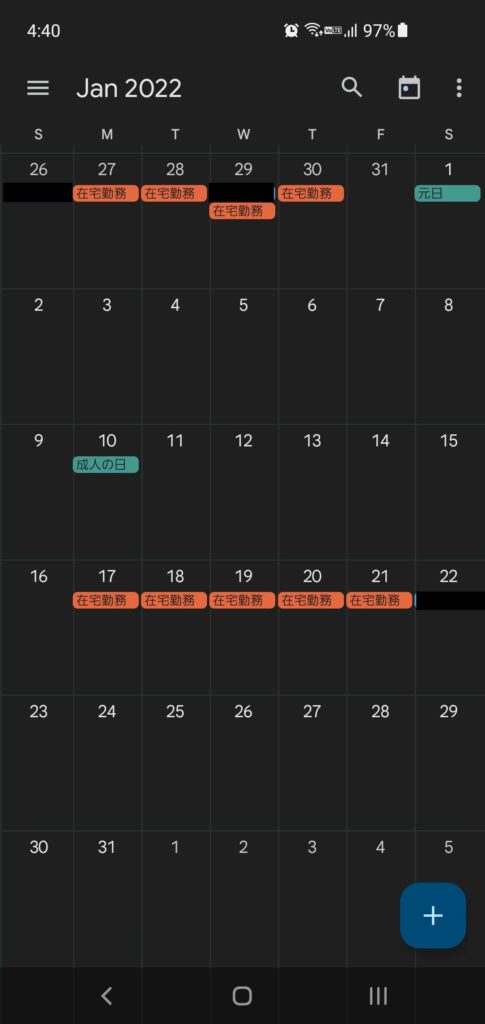
シートの造り
シートの作りとしては、基本的にシートの上の方から、値をスクリプト内の変数に設定していっています。スクリプトの流れがそんな感じになっています。「毎日入力(始まり)」から「毎日入力(終わり)」の間の値を利用しています。
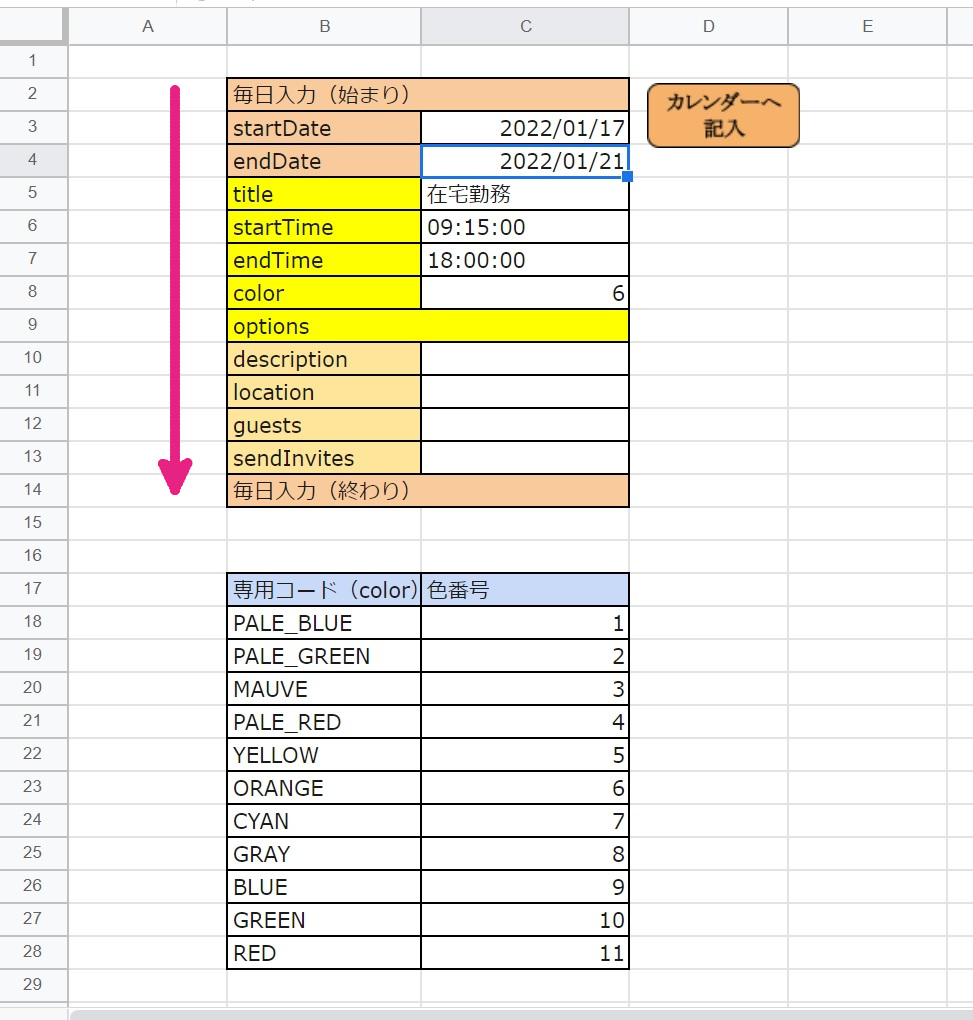
スクリプトの紹介
このツールに使用しているGoogle Apps Scriptのソースになります。
カレンダーへの入力用シート.gs
// カレンダーに予定を追加する。
function createEvents() {
// カレンダーのインスタンスを設定する。
const calendar = CalendarApp.getDefaultCalendar();
// スプレッドシートのインスタンスを設定する。
const ss = SpreadsheetApp.getActiveSpreadsheet();
const sheet = ss.getSheetByName('カレンダー入力');
const range = sheet.getRange('C3');
// 諸々の変数を設定する。
const column_of_key = 2; // キーの列:数値
let row = 1; // 走査の開始行:数値
let key; // キー:数値
let array_to_input = []; // 入力用:配列
let startDate; // 開始日:文字列
let endDate; // 終了日:文字列
let color; // 色番号:数値
let a_day; // とある日にち:文字列
let num_of_date; // 日数:数値
let startTime; // 開始時間:文字列
let endTime; // 終了時間:文字列
let options = {}; // 入力のオプション枠:辞書
let event; // イベント:Event
let day_of_EOM = {'01': 31, '02': 28, '03': 31,
'04': 30, '05': 31, '06': 30,
'07': 31, '08': 31, '09': 30,
'10': 31, '11': 30, '12': 31} // 月末の日付:辞書
// カレンダー入力データの起点を決定。
while(sheet.getRange(row,column_of_key).getValue() != '毎日入力(始まり)'){
row++;
}
// 「options」行まで、入力値を読み込む。
while(sheet.getRange(row,column_of_key).getValue() != 'options'){
key = sheet.getRange(row,column_of_key).getValue();
if(key == 'startDate'){
startDate = Utilities.formatDate(sheet.getRange(row,column_of_key + 1).getValue(), "Asia/Tokyo", "yyyy/MM/dd");
}
if(key === 'endDate'){
endDate = Utilities.formatDate(sheet.getRange(row,column_of_key + 1).getValue(), "Asia/Tokyo", "yyyy/MM/dd");
}
if(key === 'title'){
array_to_input[0] = sheet.getRange(row,column_of_key + 1).getValue();
}
if(key === 'startTime'){
startTime = sheet.getRange(row,column_of_key + 1).getValue();
}
if(key === 'endTime'){
endTime = sheet.getRange(row,column_of_key + 1).getValue();
}
if(key === 'color'){
color = Number(sheet.getRange(row,column_of_key + 1).getValue());
}
row++;
}
// 日数を設定する。(開始月と終了月が異なるかどうかで場合分け。閏年には非対応。)
if (endDate.slice(5, 7) === startDate.slice(5, 7)) {
num_of_date = Number(endDate.slice(-2)) - Number(startDate.slice(-2)) + 1;
} else {
num_of_date = Number(endDate.slice(-2)) + Number(day_of_EOM[startDate.slice(5, 7)]) - Number(startDate.slice(-2)) + 1;
}
// 繰り返し方を設定する。
let recurrence = CalendarApp.newRecurrence().addDailyRule().times(Number(num_of_date));
// 「option」の行をスキップ。
row++;
// 「options」内に入力する値を読み込む。
while(sheet.getRange(row,column_of_key).getValue() != '毎日入力(終わり)'){
key = sheet.getRange(row,column_of_key).getValue();
options[String(key)] = sheet.getRange(row,column_of_key + 1).getValue();
row++;
}
// 毎日入力
array_to_input[1] = new Date(startDate + " " + startTime);
array_to_input[2] = new Date(startDate + " " + endTime);
// Browser.msgBox('テスト');
event = calendar.createEventSeries(array_to_input[0], array_to_input[1], array_to_input[2], recurrence, options);
event.setColor(color);
}
繰り返す日数を計算します。11/30や12/31などで日数にブレが生じるのでday_of_EOM
を処理に噛ませます。
// 日数を設定する。(開始月と終了月が異なるかどうかで場合分け。閏年には非対応。)
if (endDate.slice(5, 7) === startDate.slice(5, 7)) {
num_of_date = Number(endDate.slice(-2)) - Number(startDate.slice(-2)) + 1;
} else {
num_of_date = Number(endDate.slice(-2)) + Number(day_of_EOM[startDate.slice(5, 7)]) - Number(startDate.slice(-2)) + 1;
}
ここで繰り返し入力の方法を設定します。
// 繰り返し方を設定する。
let recurrence = CalendarApp.newRecurrence().addDailyRule().times(Number(num_of_date));
この部分で、実際にカレンダーに入力します。calendar.createEventSeries()
で連続入力します。
// 毎日入力
array_to_input[1] = new Date(startDate + " " + startTime);
array_to_input[2] = new Date(startDate + " " + endTime);
// Browser.msgBox('テスト');
event = calendar.createEventSeries(array_to_input[0], array_to_input[1], array_to_input[2], recurrence, options);
event.setColor(color);
おしまい
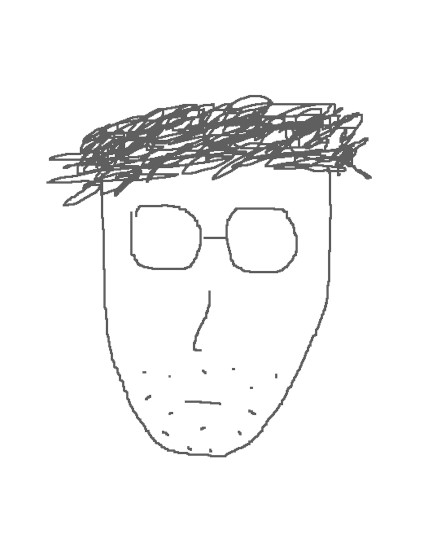
135ml
よし、これで入力した予定を1日ずついじれるようになったぜい・・・ モグモグ
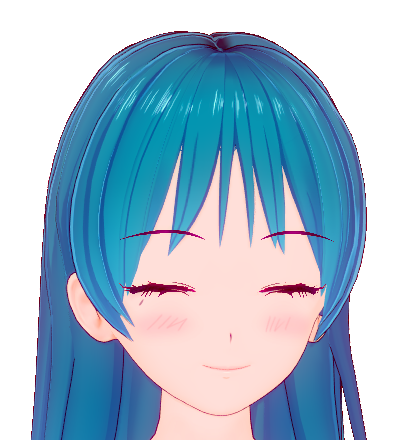
リサちゃん
ボンカレーおいしいねえ・・・ モグモグ
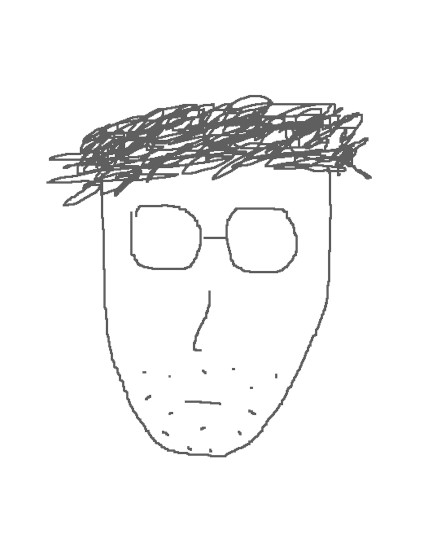
135ml
たまに食べたくなるよなあ・・・
このジャガイモが1番好きかもしれんなあ・・・ モグモグ
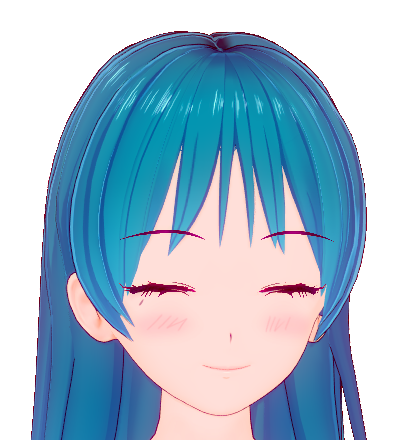
リサちゃん
また買ってくるかあ・・・
カレンダーにタスクとして入力しておこう・・・ モグモグ
おまけ
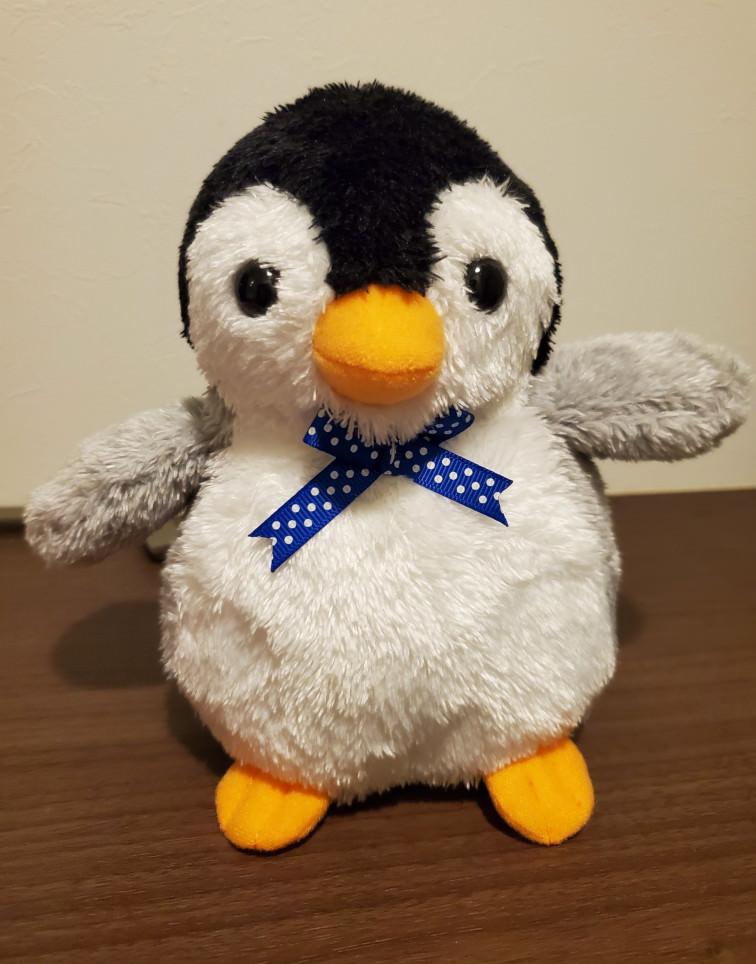
ペンギン
今回のスクリプトのソースは、このリポジトリに入っているのでよければぜひ探してみて下さい!
GitHub - landmaster135/spread-sprite-spring
Contribute to landmaster135/spread-sprite-spring development by creating an account on GitHub.
以上になります!
コメント